My maths grades were horrible. I understand the concepts, but failed at solutions in class because I’d make silly mistakes. Computers make mistakes only when they’re programmed to do so, which means maths are far more enjoyable for me as a coder. For example, take the Fibonacci sequence.
Along with prime numbers, the Fibonacci numbers are perhaps the most well-known mathematical series. Primes are:
2, 3, 5, 7, 11, 13 …
From a distance, Fibonacci numbers may look similar, though they’re not:
1, 1, 2, 3, 5, 8, 13 …
The sequence works by taking the two previous values and adding them together to make the third: 1+1=2, and then 1+2=3, 2+3=5, and so on. The values grow huge quickly.
I coded a nifty Fibonacci program for a 2017 blog post, mostly to illustrated overflow in integer values. Here is an updated version of the code, which outputs the first 100 Fibonacci values:
2020_12_12-Lesson.c
#include <stdio.h> int main() { double fibo,nacci; int count; fibo = nacci = 1.0; count=0; do { printf("%.0f\n",fibo); fibo+=nacci; printf("%.0f\n",nacci); nacci+=fibo; count+=2; } while( count < 100 ); return(0); }
The code initialized double variables fibo
and nacci
to 1.0 at Line 8. I chose the double data type as these values overflow even a long long integer size quickly.
In the do-while loop, the values are added to each other, first as fibo+nacci
at Line 14 and then as nacci+fibo
at Line 16. The results are output, and each time the values cycle to ensure that the previous two values are always added together. Variable count
is increased by 2 at Line 17 to account for the doubled-up processing.
The code outputs 100 values, each on a line by itself. The 100th Fibonacci value output is 354,224,848,179,261,997,056. While this detail is trivial, Fibonacci numbers themselves appear in nature and design because they have so many marvelous properties. O, I could wax on . . .
Another mathematical thing I’ve become fascinated with is the harmonic series. Again, this is something that would have bored me rigid in school but which I find more interesting now because I can code the problem in C and have the computer do the work.
To be nerdy, a harmonic series is the sum of a repeating cascade of values, such as the dingus shown in Figure 1. It looks pretty, but doing the math in my head is painful.
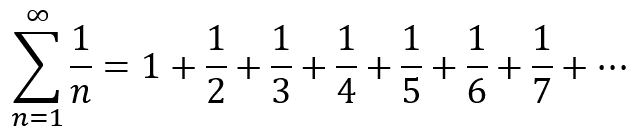
Figure 1. A harmonic series illustrated in a most frightening manner.
Like the Fibonacci series, the harmonic series comes into play with music and other things in nature that make its application more fun than the equation illustrated in Figure 1 may reveal. Of course, when I was first introduced to this concept, I looked at the equation and figured it’s something could easily code. This step was completed before I continued my study to learn about the all-important concept of “divergence.”
I cover divergence and my harmonic code in next week’s Lesson.