As you can tell by the post title, part of the sconvert program I missed is to convert tab characters into HTML spaces. Like spaces, tabs output blanks that must be converted to the
code for proper output on a web page.
In last week’s Lesson, I told the riviting story of the sconvert utility, which I use to process program output for this blog. It replaces spaces with the
(non-breakable space) HTML code. This program automates the conversion for me, so I don’t manually replace the spaces in sample output, which is a pain and takes time.
The first draft of the code, however, didn’t convert tabs. Adding tab detection and output routines requires updating the existing code. To avoid messy if-else decisions, I chose to use a switch-case structure to evaluate and convert streaming input:
2023_04_08-Lesson.c
#include <stdio.h> int main() { int ch; while(1) { ch = getchar(); if( ch==EOF ) break; switch(ch) { case ' ': printf(" "); break; case '\t': printf(" "); break; default: putchar(ch); } } return(0); }
Immediately after grabbing a character from standard input, an if test checks for the EOF
. When it floats in, the endless while loop is broken.
The switch-case structure looks for twoitems: a space, or a tab. The default condition is to output the character input, ch
. Otherwise, for the space or tab, one or four HTML non-breakable space codes (
) are output.
This update to the code converts tabs into non-breakable spaces for HTML output. One thing the code doesn’t do is to calculate tab offsets.
For example, I wrote a program that outputs the days of the week followed by a number value. Figure 1 shows the output as it appears on the screen:
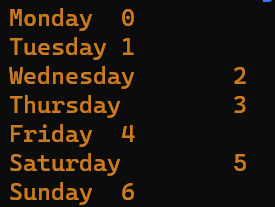
Figure 1. Text output with tabs may not line up perfectly. (Tab stops set every eight positions.)
Yes, the program output is ugly because tab stops in the terminal are set every eight column positions. Here is how the updated sconvert program interprets the output:
Monday 0
Tuesday 1
Wednesday 2
Thursday 3
Friday 4
Saturday 5
Sunday 6
Oops, the results are different. The program merely translates tabs into non-breakable HTML space codes without consideration for actual tab stops on the terminal. To more accurately convert tabs, more manipulation is required in the code, which I cover in next week’s Lesson.